C# 取得 Windows 內所有程序視窗名稱、類別、指標
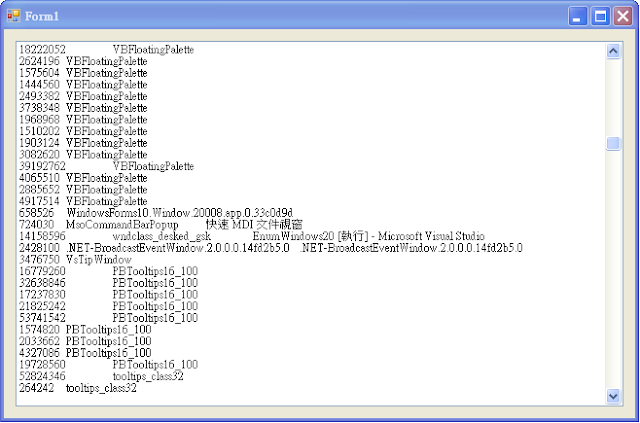
Get windows process handle / title name / class name 這篇其實是用來輔助 powerbuilder 寫的,因為大多數的第三方軟體都習慣會透過 FindWindow 這個API來確認己方程式是否已經啟動。 但是通常會不清楚自己開發工具開發的視窗會是哪種 Class Name ,所以利用下面簡單方式把電腦內所有程序的 handle / title name / class name 全部列出來查個清楚。 由於呼叫了 C 元件(COM API)所以需要多使用下面幾種 引用 using System.Runtime.InteropServices; using System.Text; using System.Collections; 程式的部分我將使用一個多行的 TextBox 把資訊列出來: namespace EnumWindows20 { public partial class Form1 : Form { //Define Unmanaged API public delegate bool EnumedWindow ( IntPtr handleWindow, ArrayList handles); [ DllImport ( "user32.dll" , CharSet = CharSet.Auto, SetLastError = true)] [return: MarshalAs(UnmanagedType.Bool)] public static extern bool EnumWindows ( EnumedWindow lpEnumFunc, ArrayList lParam); [ DllImport ( "user32.dll" , SetLastError = true, CharSet = CharSet.Auto)] public static extern int GetClassName ( IntPtr hWnd, StringBuilder lpCl